 |
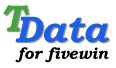
What is TData?
TData is a subclass of Fivewin's TDatabase class that provides significantly
enhanced features.
Why Use a Database Class?
A database class makes programming much easier. I could write a book on
"why," but here are a few reasons.
- You don't have to use aliases. Instead of customer->phone you use oCustomer:phone. Ok, this doesn't seem easier, but you can make oCustomer a local and use the same syntax in many routines. If you use alias referencing instead, aliases are global so you have to create a new alias for each copy of the database. This makes it very difficult to write generic routines.
- A database class provides complete buffering so you don't have to hardcode scatter and gather routines. This is all handled automatically. The data in the fieldname reference is actually in a buffer array so it can be modified without changing the data in the file. Thus you can do:
redefine get oCustomer:phone ID 101
The data oCustomer:phone can be changed. If the user then cancels the edit, the original data in the file is left untouched. Otherwise you just issue a save() and the data in the file is replaced by the data in the array.
redefine button ID 1 action (oCustomer:save(), oDlg:end())
The above line will save all the buffer data if the user presses the button. Note that TData also automatically handles locking/unlocking and automatic retries if the file is busy.
- You can add methods which can do all kinds of things. For instance, you could add a name() method to a customer class that would return the customer's full name based on first and last names that are stored in different fields.
method name()
return trim(::first)+" "+::last
These methods could, of course, be extremely complex even looking up data in other files. The big advantage of these methods is that they can be passed around with the data in the database object. So, for example, if you have a customer object with
an edit() method, you can pass oCustomer to any routine then call oCustomer:edit() and get the edit dialog.
- Polymorphism allows you to write generic routines. Polymorphism just means that you can use the same names for fields and methods. Thus you can pass a database object to a routine and call a generic method:
function myRoutine( oObject )
oObject:edit()
So, you could pass a customer object, or a vendor object, or whatever, and then call the edit method.
For more reasons to use database objects, see my articles on object-oriented programming.
Features of TData that TDatabase Does Not Have
- Selects a new workarea and opens the database with a unique alias. This is completely transparent--you never need to use aliases.
This allows you to open multiple copies of the same file using the same syntax.
- When attempting a lock, TData provides automatic retries if file or record is already locked. User can either retry or cancel. Programmer can set the number of seconds for the retry.
- Automatic retries for use() method also.
- No need to lock a record before a save. TData checks to see if the record/file is locked, if so it just does the save and leaves the record/file locked, otherwise it locks the record, saves, and unlocks it.
- Automatic locking for append() and delete() methods also.
- Provides an optional automatic progress meter when creating indexes.
- New exported methods:
setFilter() - set a filter
clearFilter() - clear any existing filter
reindex() - reindex all indexes
locked() - is locked
- New exported data:
cFile - database file name
aFieldnames - fieldnames
lShared - is shared
lReadonly - is readonly
- Works with NTXs, Comix, ADS, Harbour. TComix class included with purchase.
- Complete documentation, examples, and tutorials
TData will save you hundreds of hours of programming time, your code will be
easier to read, your programs will be more stable, and your productivity will
soar.
Copyright
2002 Intellitech. All Rights Reserved. |
|